Remove Action¶
Remove Action describes a step of the procedure which user has to remove an object using his hands or a tool.
Example of Remove Action Script:
public class RemoveJarWithToolExample : RemoveAction {
/// <summary>
/// Initialize() method overrides base.Initialize and sets the removable prefab
/// </summary>
public override void Initialize()
{
//Sets removable prefabs
//Each method call adds a new removable prefab to remove prefab list
//Ehen user removes all of them then the Action performs
SetRemovePrefab("Lesson1/Stage0/Action1/MinoanJar1RemovePivot");
SetRemovePrefab("Lesson1/Stage0/Action1/MinoanJar2RemovePivot");
SetRemovePrefab("Lesson1/Stage0/Action1/MinoanJar3RemovePivot");
SetRemovePrefab("Lesson1/Stage0/Action1/MinoanJar4RemovePivot");
SetHoloObject("Lesson1/Stage0/Action1/Hologram/HologramL1S0A1");
base.Initialize();
}
}
Action Script Explanation¶
SetRemovePrefab(string arg1)
This method sets the Action’s removable prefabs to initialize the Action behavior. To set a Remove prefab you need a string which contains the path to the removable prefab. This method can be called many times in an Action Script. Each time SetRemovePrefab is called a new removable prefab is added into the remove prefabs List. To perform the Action user needs to remove all of them.
SetHoloObject(string arg1)
Usually a remove Action does not have a hologram. Instead, we use a flashing indicator at the removable object. To enable the flashing functionality you need to check the “Attach Prefab Spawn Notifier” option at the Interactable Prefab Constructor script.
Prefab Constructor
To generate a removable prefab you need to select the “Interactable” option at the prefab constructor script. To enable the Remove Action functionality for this object you must select the “Remove” option in the Prefab Interactable serializable field at Prefab constructor script.
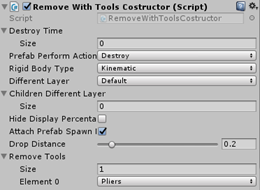
Adding More to it¶
A more advanced example is the following:
/// <summary>
/// Initialize() is the only method from basePrototype that we MUST override
/// This method initializes the Action by setting the paths to the spawned prefabs
/// </summary>
public override void Initialize()
{
//This method sets the prefab that will be removed
SetRemovePrefab("Lesson1/Stage0/Action0/MinoanJarRemove");
//Sets hologram
SetHoloObject("Lesson1/Stage0/Action0/Hologram/HologramL1S0A0Hand");
//Set Voice Actor to play after performing the Action
SetPerformAction(() => { VoiceActor.PlayVoiceActor("excellent"); });
base.Initialize();
}