Replaying Recordings¶
How Replaying accesses Recording Files¶
All Replay Players are controlled by the Component Replay in the Introduction. The Component accesses all files of the Recording and manipulates the Replay Avatars, Interacted Objects and the Scene Graph of the Operation in order to fully re-create the recorded session.
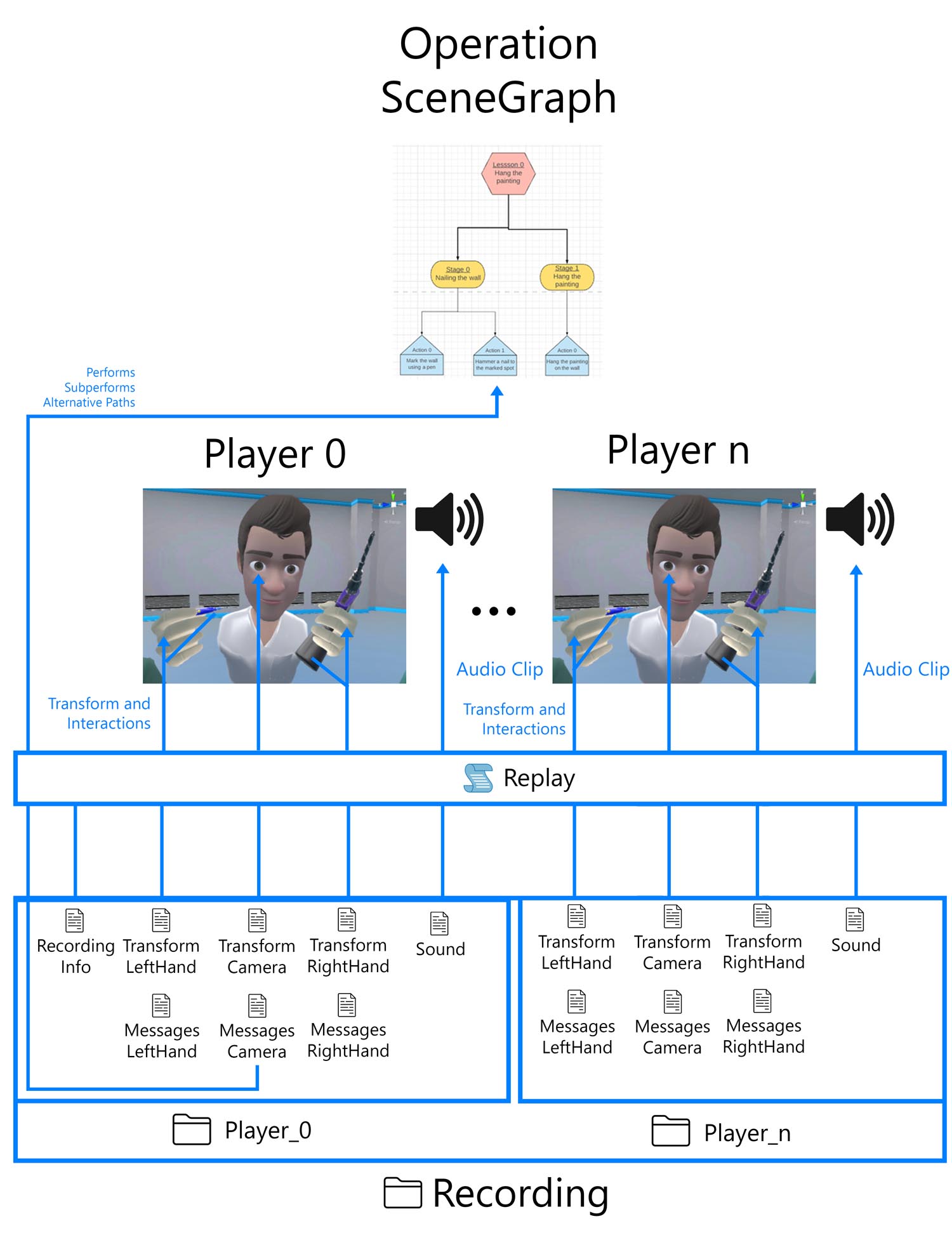
The Replay component has references to all replay avatars and fully controls them during the Replaying of an Operation. It also accesses all interacted GameObjects via name in the scene hierarchy, and creates references to them to manipulate their transform and state if they are interactables or tools.
The Sound is accessed at the beginning of the replay and formed into an audio clip that is then played back using an Audio Source. The Audio is spatial and originates from the head of the player’s avatars. The sound is also manipulated by the Replay script to match the current frame rate of the replay, that might not match the frame rate of the recording.
If a user interacts with a tool or a pump interactable, a special replaying script is placed on it, that assists in replaying interactions correctly. These scripts are placed automatically when the Replay Component detects interaction with a pump interactable or a tool. These scripts then reference the Replay script and change their state according to the recorded input that is being replayed (button presses).
Replaying interactions that require user input¶
Replaying works out of the box for Interactables and all Tools, however in order to add the specialized functionality for Interactables that require user input (E.g. Trigger Pulls), a Replaying Component must be created and attached on all applicable objects.
Warning
Tools’ states are manipulated on or off, however animations/changes related to the toggling of their state are not invoked.
1 2 3 4 5 6 7 8 9 10 11 | if (Replay.Instance.isActiveAndEnabled &&
GetComponent<OvidVRInteractableItem>().IsAttached)
{
int playerID = int.Parse(interactableItem.AttachedHand.name.Substring(19));
bool isButtonPressed = interactableItem.AttachedHand.IsLeft ?
Replay.Instance.ReplayPlayers[playerID].leftButtonsPressed[buttonName] :
Replay.Instance.ReplayPlayers[playerID].rightButtonsPressed[buttonName];
// Your functionality
}
|
Code block explanation:
OvidVRControllerClass.OvidVRControllerButtons
Using this snippet you can replicate all your interactions in Replay mode (Or even add to them).