Portal¶
Introduction¶
The Web Portal is a Single Page web Application (SPA) which combines all operations from Login and AnalyticsAPI, in an elegant UI.
Based on Authorization levels, the Portal gives Admins a wide overview of their operations regarding Licensing, Products, User Management, etc.
On behalf of Supervisors and Users, the Portal provides an overview of their analytics, scores, sessions etc.
In addition, there are pages that are not restricted on access level, such as the Profile page where users can change their password, modify their name and country, or the Dashboard.
Sample App¶
Portal comes bundled as an Angular 9+, web application.
In addition, we utilize the open-source NGX-Admin and Nebular UI kit from Akveo, for the default styling and components they provide.
Here is an example of the Portal dashboard:
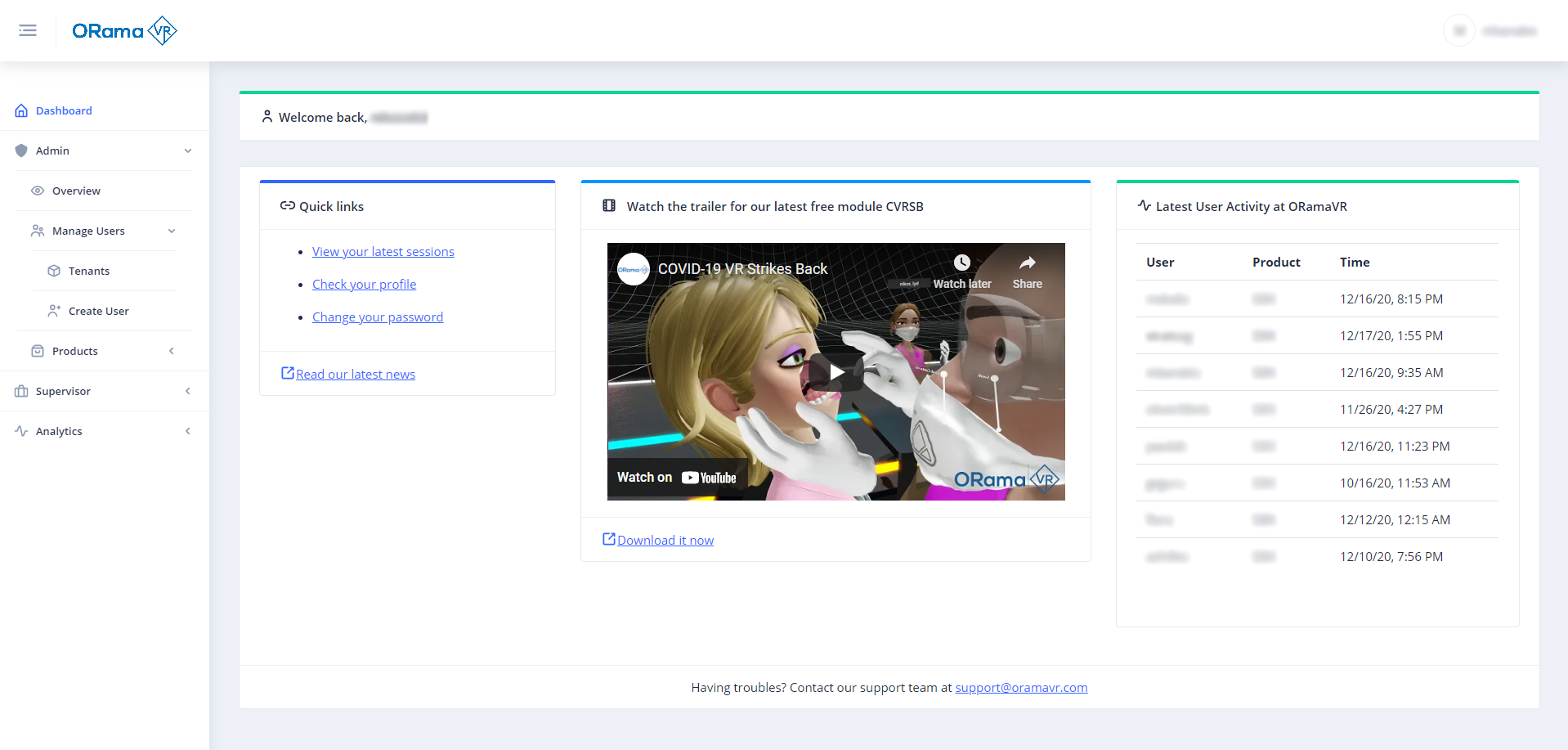
Getting Ready for Development¶
1. Angular Requirements¶
To get up and running, first you need to install Node.js, npm package manager, Angular, and the Angular CLI.
Typically, npm comes installed with Node.js, but for Node.js you have to download it and install it manually.
(Optional) Here is how to get started from the official Angular documentation.
Otherwise, follow the steps below:
Proceed to download an LTS version of Node.js.
After you finish installing, open up a terminal window and type the following command:
npm -v
(This will depict whether you have npm client installed!)Finally, proceed to install Angular CLI with the following command:
npm install -g @angular/cli
2. Node Modules¶
After Angular CLI is successfully installed, you can cd
to the directory the Portal (AnalyticsClient) is located.
There, (at the root folder) you need to type the following command:
npm install
This will create a folder named node_modules
where all third party packages and dependencies are stored.
The dependencies are specified inside the file package.json
in the root directory.
Warning
Do not source control node_modules
directory! Each developer should have his own local copy of the packages!
3. Environment Variables¶
Assuming all packages are downloaded correctly, you need to specify certain environment variables.
For the development environment, open the file environment.ts
under src/environments
.
export const environment = {
production: false,
identityServer: 'https://localhost:44355',
analyticsAPI: 'http://localhost:5002',
clientId: '',
scopes: '',
responseType: '',
};
There you need to place the localhost Login service URL, and the same for the AnalyticsAPI.
Additionally, you need to specify the IdentityServer4 Client required for the Portal to authorize with the Login service.
If you haven’t made any changes to the Login service and are running with the default Clients,
you can find this there at the Config.cs
.
In any case, here is the default configuration:
export const environment = {
production: false,
identityServer: 'https://localhost:44355',
analyticsAPI: 'http://localhost:5002',
clientId: 'WebPortal',
scopes: 'openid profile roles IdentityServerApi',
responseType: 'code',
};
4. Run the Service¶
With these minimum configuration requirements, we are ready to start the service and work localhost.
From the root directory, type the following command:
npm start
Note
It might take a while to compile the first-time, but changes you make have hot-reloading, so there shouldn’t be long waiting times.
After compilation is finished, point your browser at localhost:4200
and you are ready to go.
Keep in mind the warning below!
Warning
Login service needs to be also running, since you will redirect to it for Authentication!
5. Linting¶
By default, and to maintain a uniform coding style, we have linting in our codebase that comes from the Akveo theme.
For instance, to prevent developers from commiting console.log()
calls by mistake.
This is configured in tslint.json
. You can either discard it completely, alter the rules to suite your needs,
or keep it like this.
If you decide to stick with it, linting will prevent you from pushing to a repository unless all rules match the codebase.
Therefore, before commiting your changes make sure to run:
npm run lint:ci
This command will scan all TypeScript and SCSS files and will return errors, if any. Fix them, and then you can push ;).
Note
Other similar commands to the one above are under the file package.json
under the section scripts
. Make sure to check them out.
Getting Ready for Production¶
1. Environment Variables¶
In a similar manner to development environment, now you have to set up the environment variables for production.
For the production environment, open the file environment.prod.ts
under src/environments
.
And modify it as in the example below:
export const environment = {
production: true,
identityServer: 'https://login.yourdomain.com',
analyticsAPI: 'https://api.yourdomain.com',
clientId: 'yourclient',
scopes: 'openid profile roles IdentityServer4',
responseType: 'code',
};
2. Compilation¶
You can read all about Angular compilation in the official documentation.
In general, you need to compile Angular into an SPA.
To do so, type the command below:
ng build --prod
The above command will make use of the production environment variables you configured in the previous section, and will produce all the files you need for deployment
under the dist/
directory.
3. Deployment¶
Angular compiles into a SPA and produces one single index.html.
Because of this, you have plenty of options when it comes to deployment.
You can choose to deploy it with a simple static HTML server.
All you need to do based on the Server you are using is to configure fallback to index.html as described in the link provided.