Insert Action¶
Insert Action is referring to a specific type of Action that a user has to insert an object to a specific position in order to complete it.
For instance, an insert action can be scripted as follows:
public class PolyethyleneTrialAction : InsertAction
{
public override void Initialize()
{
SetInsertPrefab("Lesson7/Stage2/Action0/Polyethylene",
"Lesson7/Stage2/Action0/PolyethyleneFinal");
SetHoloObject(""Lesson7/Stage2/Action0/Hologram/HologramL7S2A0");
base.Initialize();
}
}
Note
Notice how the developer defined action inherits from the InsertAction base prototype.
Action Script Explanation¶
SetInsertPrefab(string arg1, string2)
This method sets the Action’s insert prefabs that will be spawned on Initialize. To set an insert Action you need to spawn two different objects, the interactable item and the final prefab. The first argument is the path to the interactable prefab while the second is the path to the final.
SetHoloObject(string arg1)
The Hologram is set for initialization through the SetHoloObject function.
Prefab Constructor
To create the correct prefabs you need to set the prefab constructors as follows.
For the Interactable prefab select the “Interactable” prefab Type on prefab Constructor script. This option will load all the necessary scripts to your interactable prefab. Then you need to check the interactable prefab constructor script and customize the serializable settings for your Action. To enable the Insert Action functionality for this object you must select the “Insert” option in the Prefab Interactable serializable field at Prefab constructor script. Next on the list is the final prefab. To generate a final prefab you need to select the “Interactable Final Placement” option from the prefab constructor script.
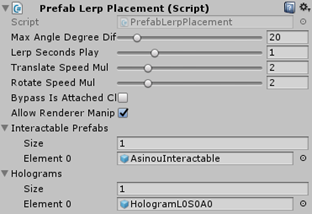
Finally, the base.Initialize() method needs to be called to set the prefabs on the BasePrototype.
Adding More to it¶
A more complex example that involves two insert actions as sub-actions is the following:
public override void Initialize()
{
AnalyticsManager.AddScoringFactor<ForceScoringFactor>(2);
//InsertAction sub-Action
InsertAction insertFrontGateAction = gameObject.AddComponent<InsertAction>();
insertFrontGateAction.SetInsertPrefab("Lesson0/Stage1/Action0/FrontPartInteractable",
"Lesson0/Stage1/Action0/FrontPartFinal");
insertFrontGateAction.SetHoloObject("Lesson0/Stage1/Action0/Hologram/FrontPartHologram");
//--------------------------------------------------------------------------------------------
//InsertAction sub - Action
InsertAction insertBackGateAction = gameObject.AddComponent<InsertAction>();
insertBackGateAction.SetInsertPrefab("Lesson0/Stage1/Action0/BackPartInteractable",
"Lesson0/Stage1/Action0/BackPartFinal");
insertBackGateAction.SetHoloObject("Lesson0/Stage1/Action0/Hologram/BackPartHologram");
//--------------------------------------------------------------------------------------------
//ToolAction sub - Action
ToolAction hitWithMallet = gameObject.AddComponent<ToolAction>();
hitWithMallet.SetToolActionPrefab("Lesson0/Stage1/Action0/BackPartHitMallet", MAGES.ToolManager.tool.ToolsEnum.Mallet);
hitWithMallet.SetHoloObject("Lesson0/Stage1/Action0/Hologram/MalletHologramL0S1A0");
InsertIActions(insertFrontGateAction, insertBackGateAction, hitWithMallet);
base.Initialize();
}
In the above example, notice how each individual insert action follows the exact same pattern of object initialization.