Optional Action¶
In this tutorial we will learn how to compose an Optional Action and make our applications more interesting.
Note
Optional Actions can be used as side-tasks, Actions that are not mandatory to be performed.
The scenario¶
In this example our main Action will be a QuestionAction, asking the user where is Sponza located. At the same time we would like for a second Action to be spawned. This Action involves the insertion of a jar into a predefined place (InsertAction). After inserting the jar we would also like to hammer it into place (ToolAction). Those two Actions would be in a lesson.
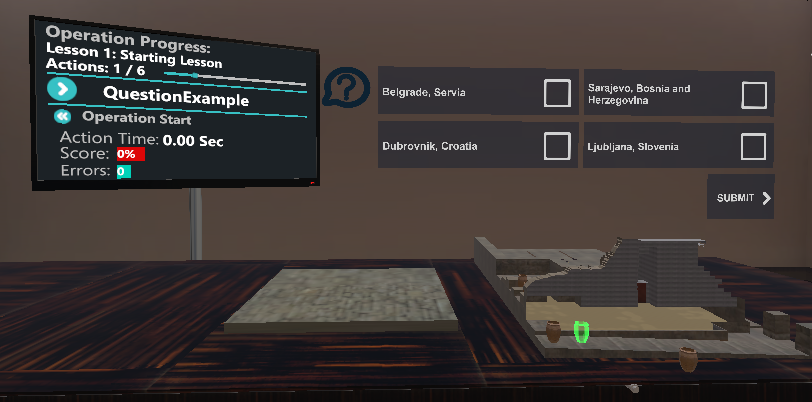
Optional Action Implementation¶
The implementation of the Actions is simple and does no different from implementing a simple Insert and a Tool Actions.
Insert the Jar (Insert Action)
using MAGES.ActionPrototypes;
public class InsertJarAction : InsertAction
{
public override void Initialize()
{
SetInsertPrefab("Optional/InsertJar/Action0/JarInteractable", "Optional/InsertJar/Action0/JarFinal");
SetHoloObject("Optional/InsertJar/Action0/JarHologram");
base.Initialize();
}
}
Hammer the Jar (Tool Action)
using MAGES.ActionPrototypes;
public class HammerJarAction : ToolAction
{
public override void Initialize()
{
SetToolActionPrefab("Optional/InsertJar/Action1/JarHitMallet", MAGES.ToolManager.tool.ToolsEnum.Mallet);
SetHoloObject("Optional/InsertJar/Action1/JarMalletHologram");
base.Initialize();
}
}
Add Action to scenegraph¶
Now that we have the two Actions it is time to add them to scenegraph.
Open the existing scenegraph tree and construct a lesson with a stage containing the above two Actions. You can reconstruct the Optional Lesson as you want, in our case we included both Actions into a single Stage.
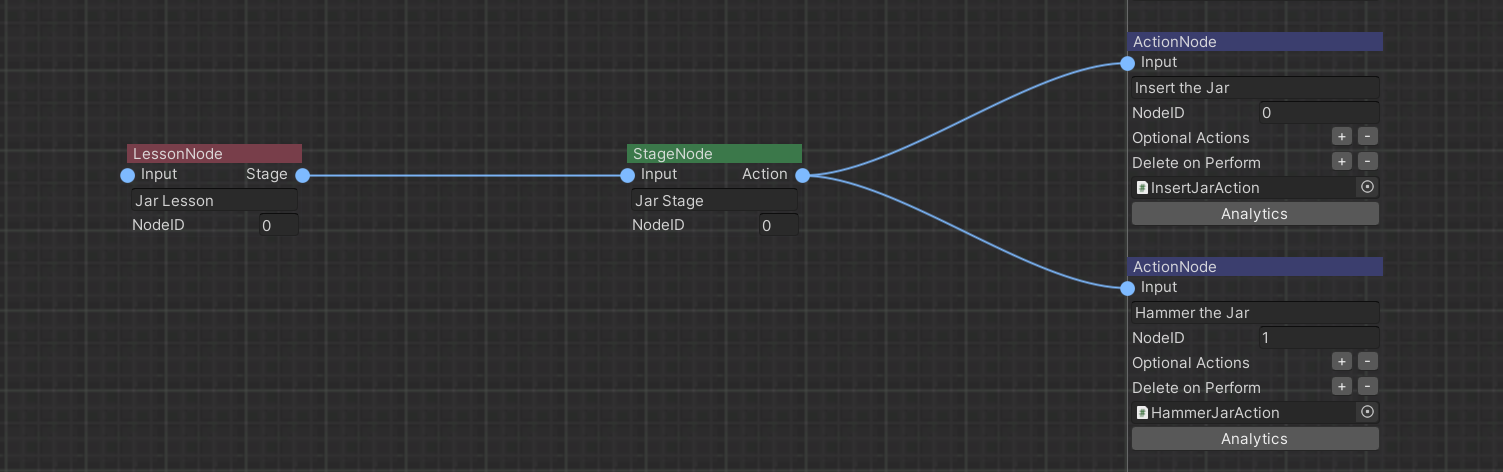
Warning
Pay attention that didn’t link the Lesson we created with the operation node. This is because this Lesson is not a part of the scenegraph’s main path. It is an optional Lesson.
To make the Lesson Optional, right click on the lesson node and select “Set Optional Node”
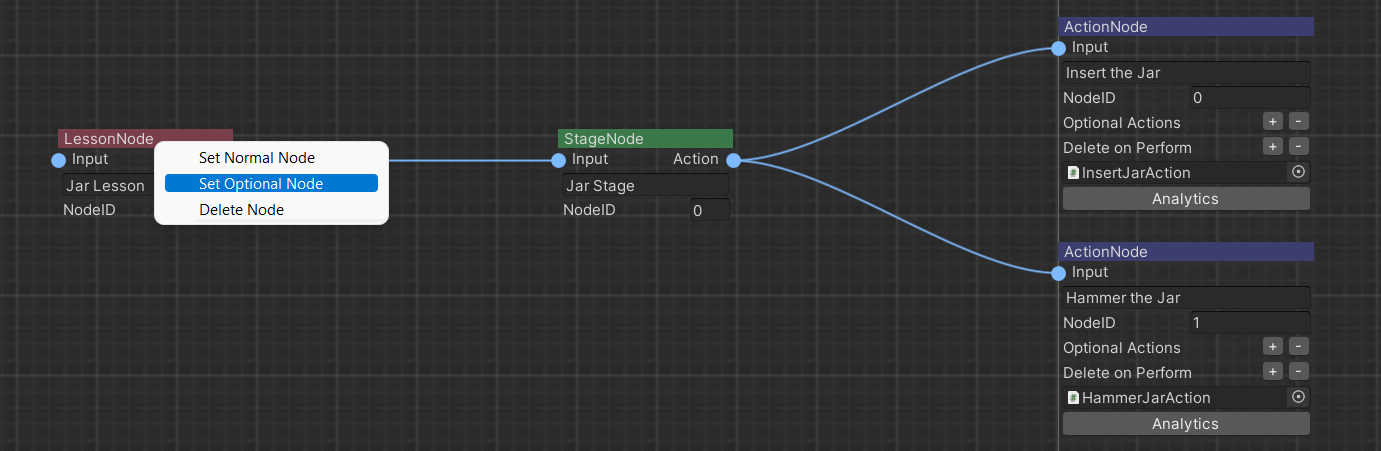
This will make all the nodes contained into the Lesson Optional Nodes. A tag “Optional” is also written into the node labels.
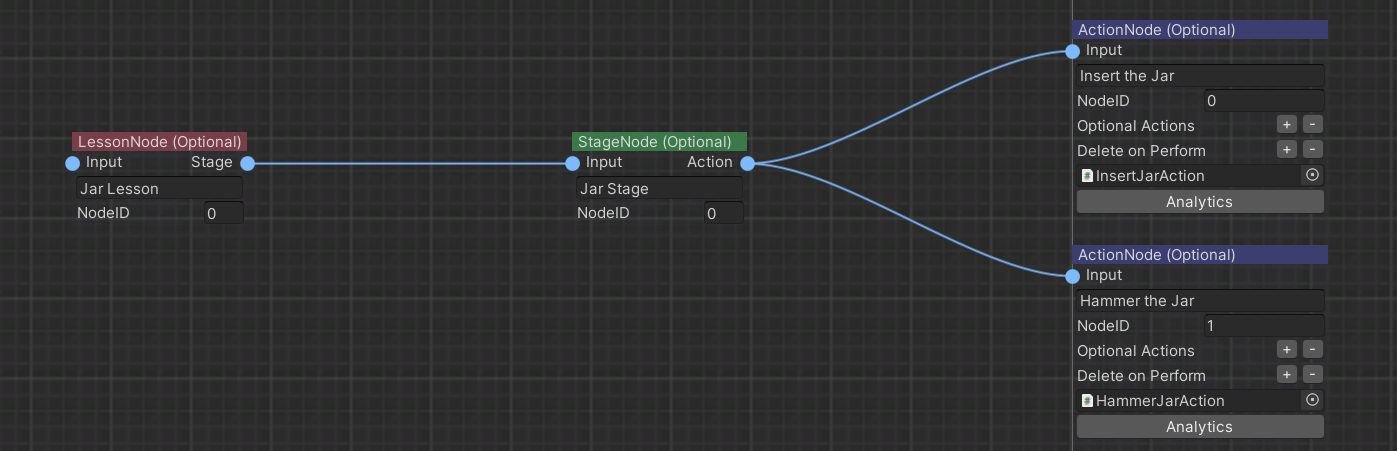
The next step is to set where this Lesson will be spawned. As mentioned before we will spawn it with our QuestionActions as an Optional.
To do that we will go to the QuestionExample and add type the Jar Lesson at the optional Actions List. You can instantiate more than one Optional Lessons. This will instantiate the first Action of the Jar Lesson along with the QuestionExample Action
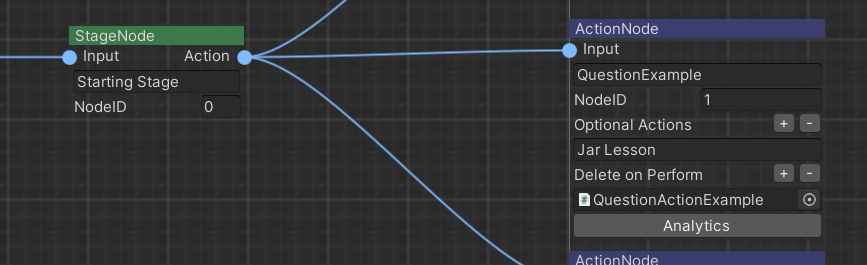
We also want to set when this Optional Lesson will be deleted. In our case we want to delete it after the Insert the plug Action.
To do that we will go to the Insert the plug and add the Jar Lesson in the destroy on perform List. You can delete more than one Optional Lessons. By performing the Insert the plug Action we will also delete our Optional Lesson, if the user did not perform it till then.
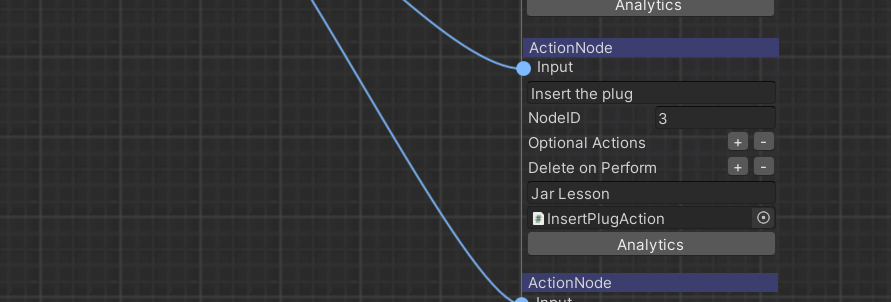
Note
In this way we configure the lifetime of our Optional Action.
Remember to save the scenegraph after this step.
Final result¶
The gif below shows the Optional Action we implemented. As you can see the InsertJarAction remains active till we decide to perform it.
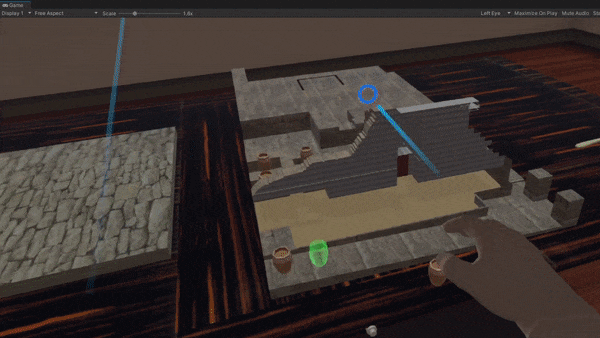
Scenegraph manipulation with Optional Actions¶
Optional Actions can also be used modify the Scenegraph tree according to the user’s decisions.
Note
This functionality can be used in cases that an Action is crucial and by performing it the scenegraph will update itself by adding or removing other Actions. An example can be a completion of a wrong Action by mistake.
In this tutorial we will demonstrate this decision-making functionality.
The scenario¶
In our case, we would like to generate a decision-making case for our users to decide whether to assemble the Knossos or the Sponza. If the user decides to assemble the Knossos (right), the scenegraph will move to the next Action. However, if he assembles the Sponza (left), we will replace the Knossos Lesson with an Optional one, the Sponza lesson.
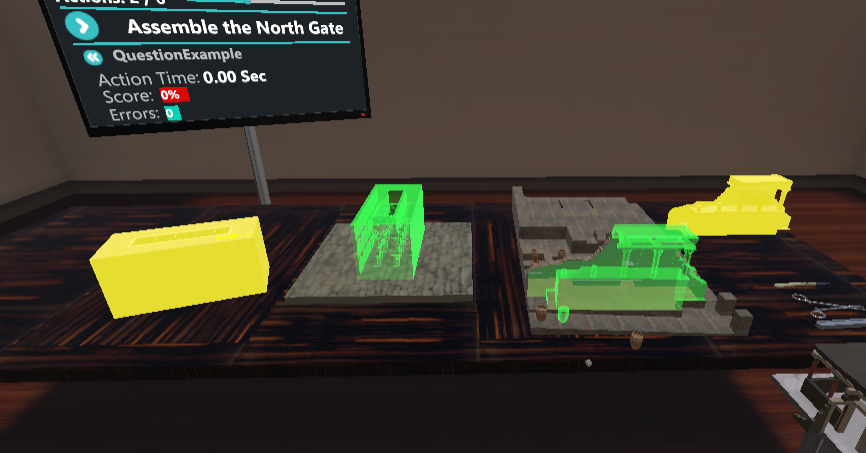
Action Scripts¶
Assemble the Knossos (Insert Action)
using MAGES.ActionPrototypes;
public class AssembleKnossosAction : InsertAction
{
public override void Initialize()
{
SetInsertPrefab("Lesson0/Stage1/Action0/FrontPartInteractable", "Lesson0/Stage1/Action0/FrontPartFinal");
SetHoloObject("Lesson0/Stage1/Action0/Hologram/FrontPartHologram");
base.Initialize();
}
}
The Knossos Action is just a simple Insert Action.
Assemble the Sponza (InsertAction)
using MAGES.ActionPrototypes;
using MAGES.sceneGraphSpace;
public class AssembleSponzaAction : InsertAction
{
public override void Initialize()
{
SetInsertPrefab("Lesson0/Stage1/Action0/SponzaInteractable", "Lesson0/Stage1/Action0/SponzaFinal");
SetHoloObject("Lesson0/Stage1/Action0/Hologram/HologramSponzaFinal");
//It is important to add this line if your perform method changes the scengergraph runtime
// (eg uses the ReplaceNoreRuntime)
GetComponent<ActionProperties>().actionType = ActionType.OptionalConvertedToNormal;
base.Initialize();
}
public override void Perform()
{
ScenegraphTraverse.ReplaceNodeRuntime("Knossos Lesson", "Sponza Restoration");
base.Perform();
}
}
This is the Optional Action, as you see it overrides the Perform method and includes some code.
The ReplaceNodeRuntime("Knossos Lesson", "Sponza Restoration")
function will replace the Knossos Lesson with the Sponza Restoration Lesson (this is an Optional Lesson) if the user Performs this Action.
Alternatively, you can use the AddNodeRuntime("Sponza Restoration")
to add the Sponza Restoration Lesson or the DeleteNodeRuntime("Knossos Lesson")
that will only delete the Knossos Lesson.
Warning
Make sure to include the line GetComponent<ActionProperties>().actionType = ActionType.OptionalConvertedToNormal;
in the initialize method if you want to Destroy the main Action and initialize the next one after performing this optional.
Scenegraph configuration¶
For this implementation we need to configure two Optional Lessons. The Optional Lesson to insert the Sponza (this will trigger the second one) and the Sponza Restoration Lesson that will replace the Knossos one in case the user decides to assemble the Sponza.
Below you see the Assembly of Sponza

And this is the Sponza Restoration Lesson
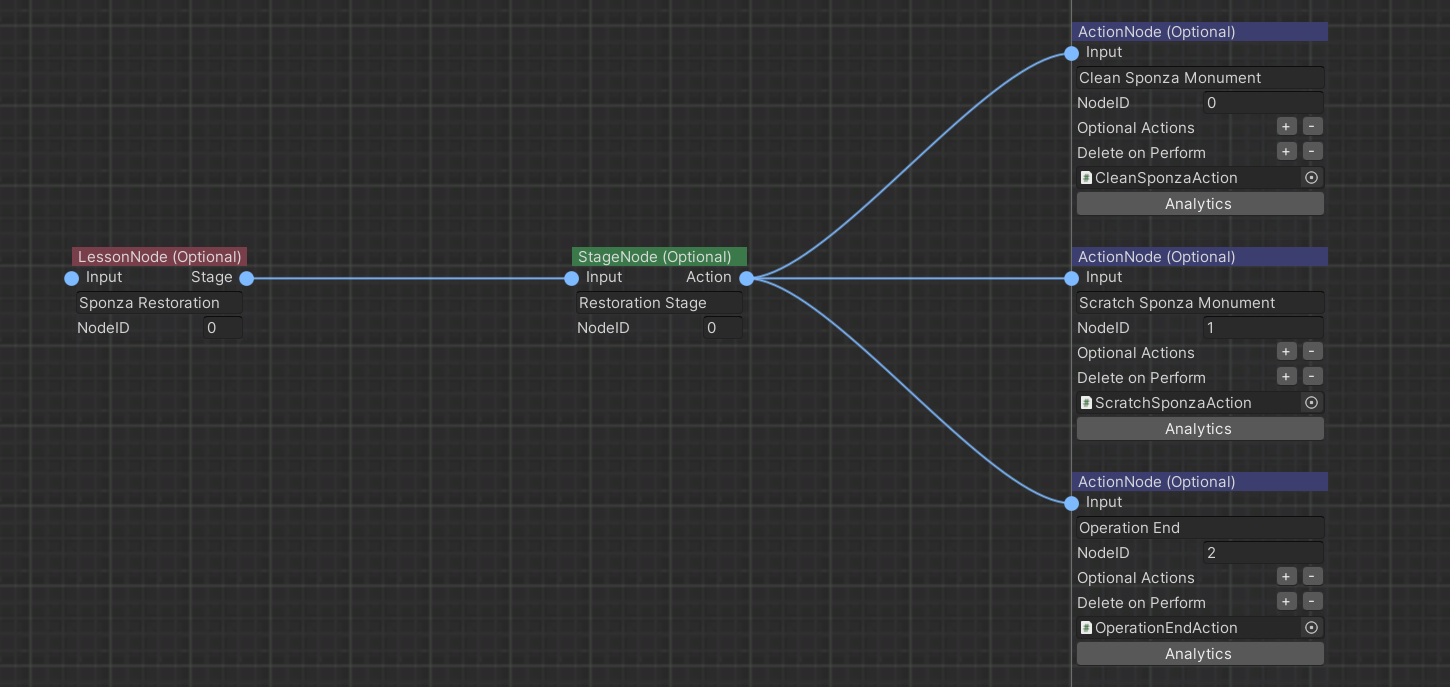
Note
Remember to mark those nodes as Optional nodes.
Finally, we have to configure the point where we spawn the Sponza Optional Action. This would be the same Action with the Knossos, to make the user decide what he will assemble.
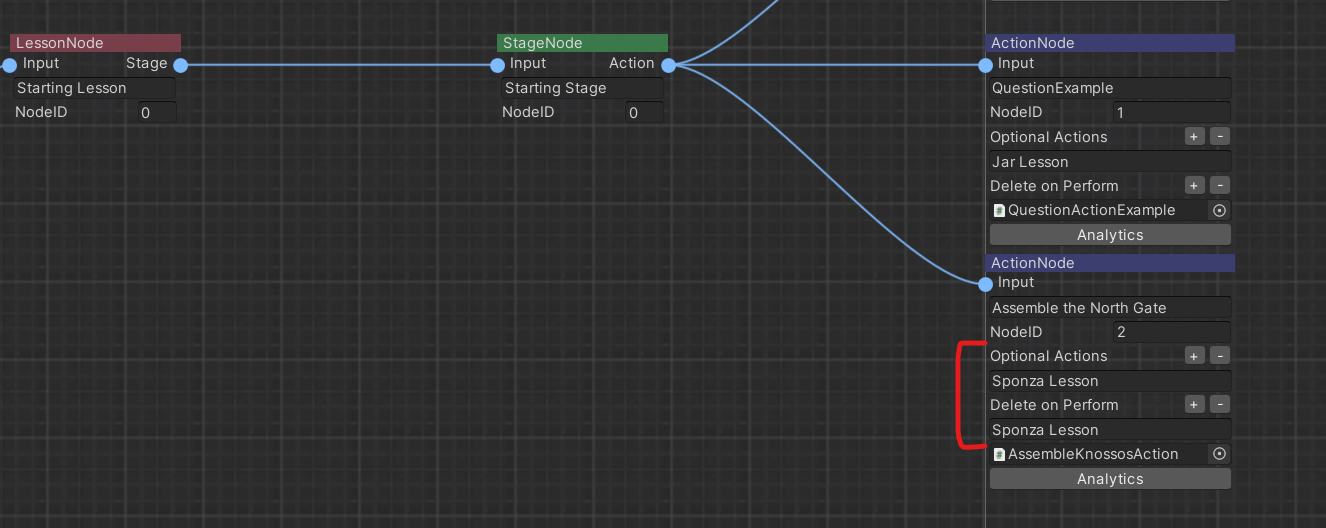
Pay attention that we will also destroy the Sponza Optional node if it is not performed after this Action.
Final result¶
The gif below shows the Optional Action we implemented. As you can see if we perform the Knossos Action we continue with the assembly of Knossos. However, if we return and do the Sponza Action, we proceed with the Sponza restoration Actions (Optional Lesson).
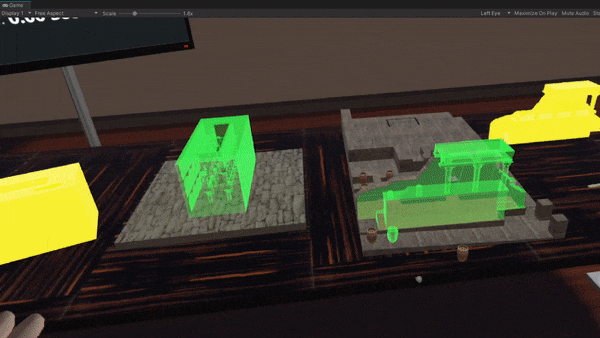