Generating Analytics¶
As discussed thoroughly in Analytics File System, Analytics expect certain assessment formatting (e.g., scoring data, accumulated user data, etc.) and produce certain output.
ORamaVR provides a simple out-of-the-box solution for generating analytics for your products. In detail, analytics are per action and have to be explicitly specified for each action in your storyboard.
Note
Recall, Actions are generated from the SceneGraph Editor.
Visual Editor¶
To specify the assesment (i.e., analytics recording) for each Action, start by opening the SceneGraph Editor through the MAGES menu.
MAGES/SceneGraph Editor
Proceed to Load the Storyboard XML by selecting File/Load and navigate under Assets/Resources/Storyboard/platform/
You will be presented with a similar view:
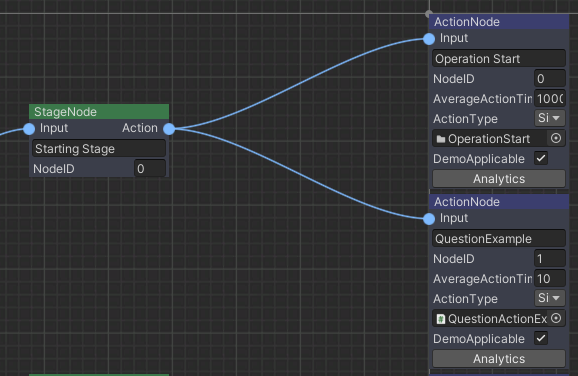
Under each Action there is an Analytics button.
Press on this button and the following window will open
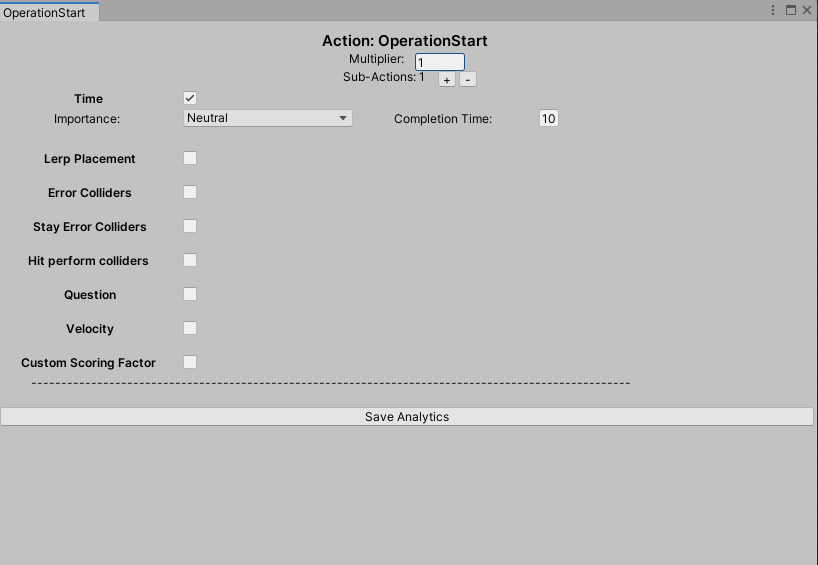
In the Analytics window you can specify all scoring factors for the current Action.
Scoring factors¶
As described in Analytics File System, MAGES SDK supports a variety of predefined scoring factors.
Current available scoring factors are enumerated below in an algorithmic manner following the order of the SceneGraph Editor:
Time
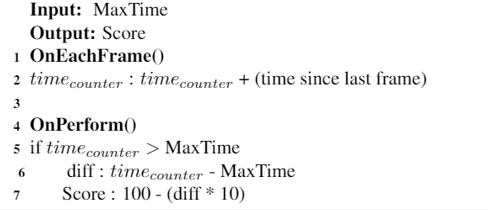
Lerp Placement
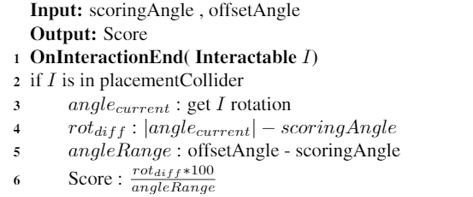
Error Colliders
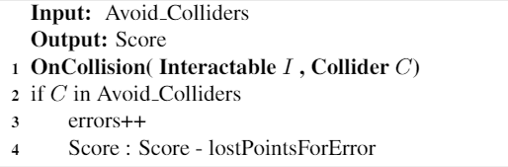
Stay Error Colliders
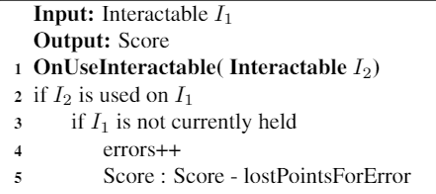
Hit Perform Colliders
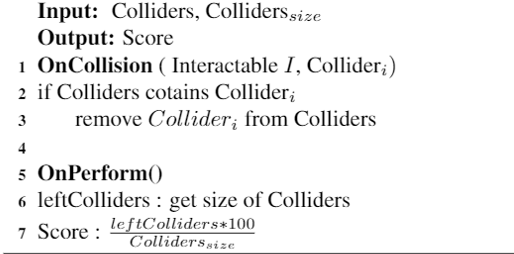
Question
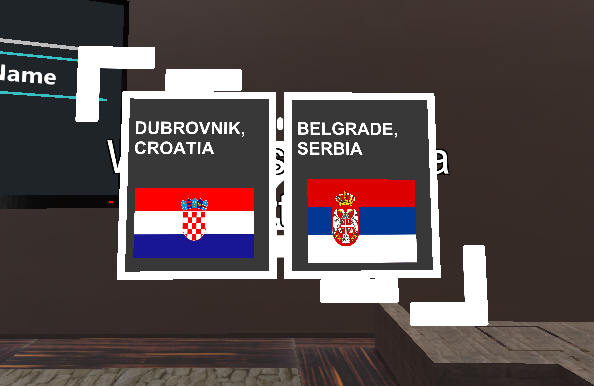
Velocity
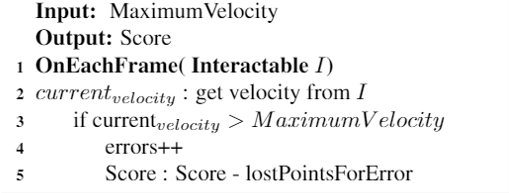
Using the above combinations you can produce an output similar to the following:
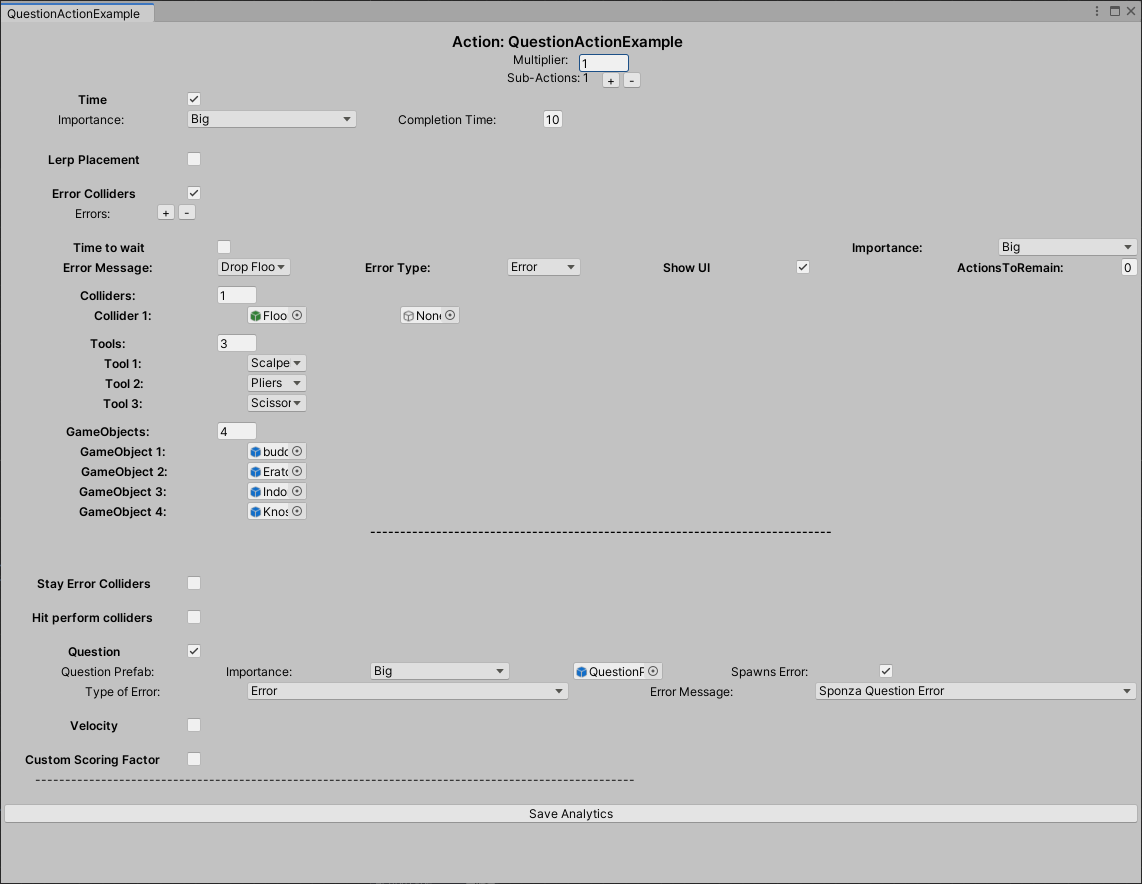
Finally, click the Save Analytics button down in the editor window to save your changes.
Warning
If you forget to Save your Analytics for each action, the changes will get discarded.
Custom Scoring Factor¶
Custom Scoring Factor is Work in Progress (WIP) and cannot be modified from the Editor. However, you can implement it directly in code.
You can implement a Custom Scoring Factor as in the SampleApp example below:
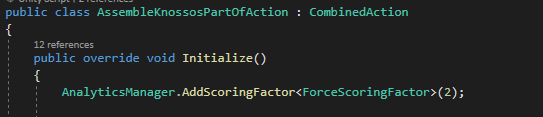
And the respective ForceScoringFactor script in code as follows:
public class ForceScoringFactor : ScoringFactor
{
public float maximumForce = 12;
UpdateCollisionForce _forceScript;
float _currentForce;
GameObject _knossosBackPart;
ForceScoringFactor _forceSf;
int _score;
LanguageTranslator _errorMsg;
public override ScoringFactor Initialize(GameObject g)
{
_forceSf = g.AddComponent<ForceScoringFactor>();
_knossosBackPart = GameObject.Find("BackPartHitMallet(Clone)");
_forceScript = _knossosBackPart.AddComponent<UpdateCollisionForce>();
_forceScript.Init(maximumForce,true);
return _forceSf;
}
public override float Perform(bool skipped = false)
{
Destroy(_forceSf);
Destroy(_forceScript);
if (skipped) return 0;
int errors = _forceScript.GetErrorsCounter();
_score = 100 - (errors * 20);
return Mathf.Clamp(_score,0,100);
}
public override void Undo()
{
_score = 0;
Destroy(_forceSf);
Destroy(_forceScript);
}
public override object GetReadableData()
{
ScoringFactorData sfData = new ScoringFactorData();
sfData.score = _score;
sfData.outOF = (int) maximumForce;
sfData.type = "Force Scoring Factor";
sfData.scoreSpecific = (int) _forceScript.GetCollisionForce();
sfData.errorMessage = InterfaceManagement.Get.GetUIMessage(_errorMsg);
return sfData;
}
}
Note
Notice how our custom scoring factor extends ScoringFactor